Most random interview asking questions in Javascript
Hi everyone,
Hope all are doing well. Today I am going to talk about interview asking questions in javascript👌👌. So let’s start…😎😎😎😎

1undefined vs null: undefined means the value of the variable has not been assigned or for any function, if there is no return value then the function returned “undefined”. Because javascript variables start with undefined if they are not initialized or defined. The type of undefined or any undeclared variable is the string “undefined”
Example:

“Null” means the variable has no value and that is known by the programmer and also value is assigned as ‘’null’ by the programmer.
Example:

2let vs var vs const: var, let & const the statement declares a variable in javascript.
var: var is a statement to declares a variable. It is a functional and global scope. It can be re-assigned.
Example:

Let: It works as the same var but it is not a global scope. (scope is another important topic in javascript. we will not cover in this article). It is a block scope. As like var, it can be re-assigned. It is one of ES6’s features.
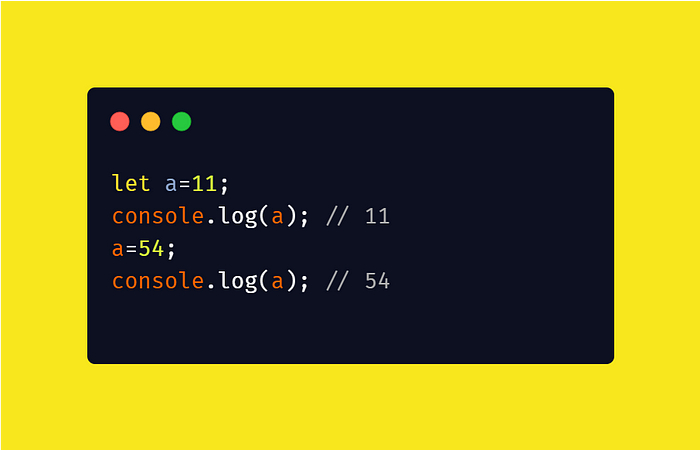
const: const is also a statement of declaring a variable. It is also an ES6’s feature. It is also a block scope. If you need a constant value of a variable you should use the const keyword. It can’t allow re-assigned variables. If we will try to re-assigned a variable with const then you should get an error like below :
TypeError: Assignment to constant variable.at Object.<anonymous> (c:\Users\Personal\Desktop\BlogsForJS\index.js:220:2)at Module._compile (internal/modules/cjs/loader.js:1063:30)at Object.Module._extensions..js (internal/modules/cjs/loader.js:1092:10)at Module.load (internal/modules/cjs/loader.js:928:32)at Function.Module._load (internal/modules/cjs/loader.js:769:14)at Function.executeUserEntryPoint [as runMain] (internal/modules/run_main.js:72:12)at internal/main/run_main_module.js:17:47
Examples:
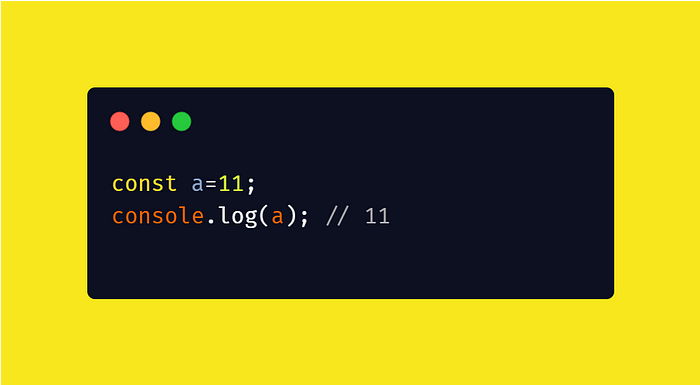
3.Double equal vs triple equal: The most important thing when we compare two variables in javascript equal or not equal. In this case we use == or ===. Both of them return a true or false value.
- == (Double equals operator): Known as the equality or abstract comparison operator. Double equal doesn’t concern about the type it is concern about value.

2.=== (Triple equals operator): Known as the identity or strict comparison operator. If two value has the same type then it returns true otherwise false.

4. Global scope in Javascript: Scope in javascript determines by the visibility of variables. There are two types of scope in javascript. global and local scope. Every javascript function creates a scope. If your variable is declared outside of the function then it's called a global variable or global scope. global variable or global scope is accessed anywhere in code.
Example :

5.Local scope in Javascript: If your variable is declared inside of a function then it’s called local variable or global scope. local variable access only the parent function of this variable. Outside the function, we can’t access the local variables. Look at the example :

If you try the below code :

then you will get this error:

Scoping idea is applied for the function and object also.
6.Closure in javascript: One of the most important topics for Javascript interview questions. Let’s explore what is it?
The closure is by default behavior in Javascript.See, we declare a function parentFunc() and inside this function we declare another function childFunc(). When we return the parentFunc() and then from childFunc() we can also access the parent function’s variable and object also. It's happening for Closure in Javascript. look at the below images.

7.Private variable in Javascript: private variable in javascript is an OOP(Object-oriented programming concepts). Which means which variable we declare as a private variable it can’t be accessible in other classes. It is only accessible in its parent class.
8.Window object in Javascript: The window object in javascript represents the global object in browsers. Global variables are properties of the window object. There are many types of the global variable exists. look at the below images.

9.What is DRY: If we can live in good health we need clean and calm food, an environment, and so on. In the same way, if our code should be clean and clean so that everyone understands the code. The DRY principle helps us to keep this type of code. DRY means Do not Repeat Yourselves. That means we write one type of code one time. Not again and again we repeat the same code.
10.IIFE: Generally when we create a function for any reason it should be called/invoked for the execution of the function. What if when we create the function and immediately call this?😉 That is the concept of IIFE. IIFE means Immediately Invoked Function Expressions. We create a function and call the function at a time.
Look at the Example:


So, this is from my side. I think these topics are very important for any interview question for the junior level. So, that’s it for today.