Introduction to REACT.JS
All You know as a new REACT.js Developer
React is a popular javascript library made by Facebook on 29th May 2013. It is not exactly a framework. If you start learning React.js then this article is for you. So, Let’s go and explore React.js from Scratch.

1.WHAT is REACT.JS: React.js is a javascript library for build an interactive user interface. It is a library, not a framework. There is a huge difference between library and framework. We do not go there exactly we will explore today the popular javascript library REACT.js.
It is a component-based library. We can break a web app into many pieces components. Then by contacting all of the components we get a full web app. React makes it easier to create an Interactive UI. It works like a function(in every programming language we use function) and it makes code drier (DRY-Don’t Repeat Yourself). Learn more.
2.WHY REACT.JS: why should we learn React.js? why not the popular library/framework in the market like Vue.js, Angular.js, Jquery, Meteor, Ember.js, Mithril, etc.

Let’s break down why I choose to React?
1.It is very simple and declarative.
2. For a better user and developer
experience
3.Supports server-side
4. Mostly it is a component-based UI library.
5. Optimizing time and code for developer
6.It is concern about changing state not UI
7. For making SPA (Single page application) and PWA(Progressive Web App)
8. Also the huge level of community support
For the above reason, I choose the REACT.JS as a 2021’s Javascript Library. Why?
3.Now we will see the core topics or concepts we should know as a React.js Developer.
- Components
- Life-cycle Methods.
- State
- Props
- Default props
- Virtual DOM
- JSX
- HOOKS the power of functional components
- State Management
4.So, now we start with Components in React: Component is one of the core building blocks in React. It’s like the lungs of a React Application. You can’t think of an application without a component. Throw the component we make an application’s Ui easily. Look at the following image and understand what is a component.

Here we can see an application on the right of the image and on the left we can see the small pieces of the entire website. The left side of the images shows the part by part of the entire application, these small pieces are called components. After connecting together they make the full application like the right side of the images.
There are two types of components that exist in React.
- Functional components.
- Class-based Components.
5. Life cycle method in React: You can think of a life-cycle method in REACT that is like a men’s life. Every component in React goes through a lifecycle of events. I like to think of them as going through a cycle of birth, growth, and death of a men’s life. Rect has 3 stages in the entire application.
Like :
- Mounting: the birth of men. (here you can replace the men with a component.)
- Updating: growing up the men.
- Unmounting: death of the men.
As men React also has the above three-stage in a components life-cycle in React. It is called the life-cycle method in React. Look at the below image.

6.State and props in react:
State: In React State is one of the core concepts you should know about this. The State of a component is an object that holds some information that may change over the lifetime of the component. The State object is where you store property values that belong to the component. When the State object changes, the component re-render. Data in the state control what you see in the UI. Look at the picture below.
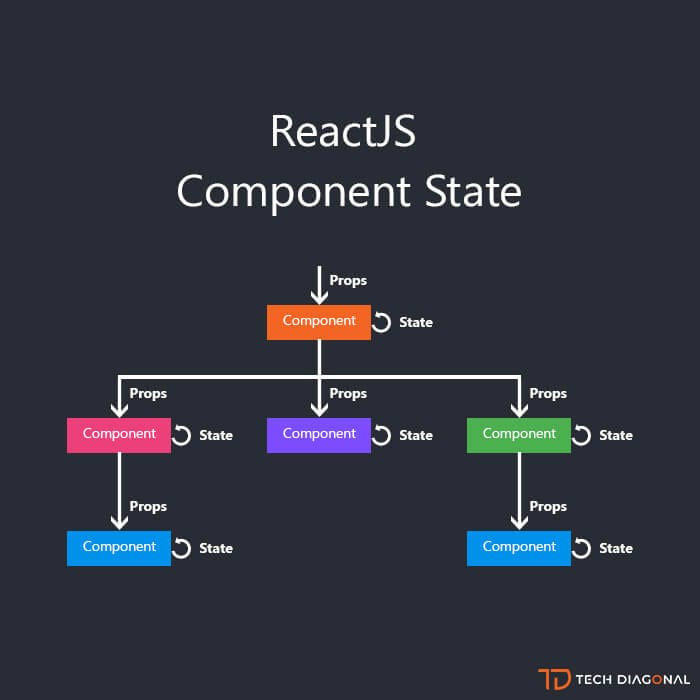
Props:Props is short for properties and they are used to pass data between React components. React’s the data flow between components is uni-directional (from parent to child only). look at the below images.

For a better understanding of props and state in React go here.
7.Default props in react: we pass data between parent component to child component using props. What if when we didn’t pass any data from parent or props is not passed? At this time the application will be crushed. For reducing this problem we use default props. That means we set a default props data.
8.Virtual DOM: React has a virtual DOM which is a copy of the actual DOM and is kept in the browser Memory in the form of a javascript object. React also has the state and props, which hold the data of the application and is used to pass that across the components in the hierarchy.
9.JSX: We all know about the Html tag. JSX is a JavaScript Extension Syntax used in React to easily write HTML and JavaScript together. Read more.
Example :
HTML:const element = <h1>Hello, world!</h1>; JSX :function formatName(user) {
return user.firstName + ' ' + user.lastName;
}
const user = {
firstName: 'Harper',
lastName: 'Perez'
};
const element = (
<h1>
Hello, {formatName(user)}! </h1>
);
ReactDOM.render(
element,
document.getElementById('root')
);
10.State Management: One of the most important concepts in React. It makes React more and more powerful. We can manage the state in three ways.
- By props. (From parent to children components)
- by Context API
- by REDUX